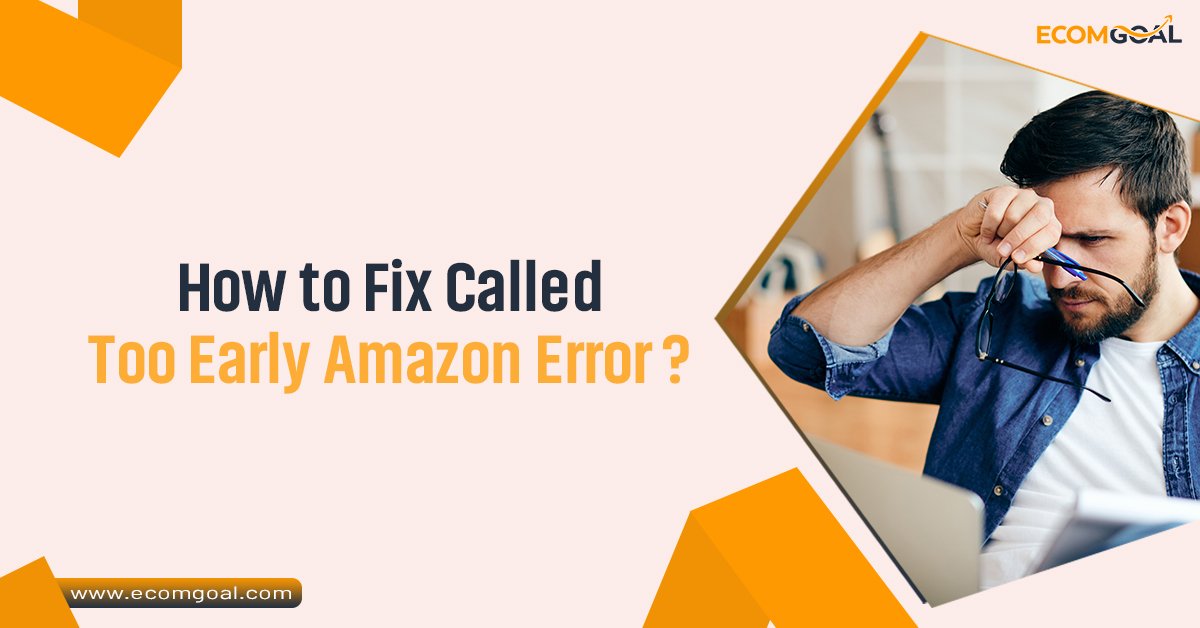
In this comprehensive guide, we’ll explain how to fix called too early Amazon error, why it happens, and provide step-by-step instructions to help you fix it and prevent it from occurring in the future.
What Is the “Called Too Early” Amazon Error?
The “Called Too Early” error on Amazon occurs when an API call is made to Amazon’s server, but the request is sent before certain prerequisites or data availability conditions have been met. This error often affects developers or sellers who use third-party integrations (via Amazon MWS or Seller APIs) to automate tasks like listing products, managing inventory, or retrieving order data.
For example, if you attempt to call a product feed submission to Amazon before the system has finished processing an earlier feed, the system might throw this error, essentially stating, “You are making a request before the required action is ready.”
Common Scenarios Leading to the “Called Too Early” Error
Several scenarios can trigger this error:
- API Rate Limitations: Amazon APIs have specific limits on how many requests can be made within a certain time frame. If you exceed the allowed rate, you might trigger the error by sending requests too quickly.
- Premature Product or Inventory Updates: If you try to update or create a new listing before the system has finished processing an earlier related action (like a product image upload or a previous feed submission), you might encounter this error.
- Order Data Retrieval Before Availability: Sometimes, sellers attempt to retrieve order or inventory data before it has been fully processed by Amazon’s system, causing a premature call.
- Dependency Failures: Some operations on Amazon are dependent on the completion of previous tasks. If those earlier tasks haven’t finished yet, the next dependent action may result in this error.
Learn More: How to Fix Error 5665 in Amazon
Step-by-Step Guide How to Fix Called Too Early Amazon Error?
1. Check API Rate Limits and Throttling
Amazon APIs, including MWS and Selling Partner APIs (SP-API), enforce rate limits to ensure system stability. These limits regulate how many requests you can make per second or minute. Exorbitant these limits will result in an error.
- Solution: Implement proper throttling in your API integration to respect the rate limits imposed by Amazon. Most APIs return information about current usage, such as remaining allowed requests within a time window. Adjust your system to include a delay or retry mechanism if you’re close to reaching the limit.
For example, the Amazon MWS feed API might allow one submission per 60 seconds. If you make another submission within that 60-second window, you could receive the “Called Too Early” error. In this case, programming a 60-second delay between submissions will resolve the issue.
2. Ensure Completion of Previous API Calls
Another common cause of the “Called Too Early” error is when sellers attempt to initiate a new action before the system has completed processing a previous one.
- Solution: Use the appropriate Amazon MWS or SP-API response to ensure the completion of the previous task before making the next API call. For example, when submitting feeds, make sure the first feed submission has been processed and completed before submitting another one.
One way to handle this is by checking the status of the submission using Amazon’s GetFeedSubmissionResult API call. This allows you to wait for the successful completion of the first task before attempting another.
3. Implement Error Handling and Retry Logic
In many cases, errors like “Called Too Early” are temporary and resolve themselves once the system has caught up with the requested operations. It’s essential to implement proper error handling and retry logic in your integration.
- Solution: Instead of stopping all processes when the error is encountered, your system should be able to catch the error, wait for a defined period (e.g., 5-10 seconds), and then retry the API call.
Example code:
python Copy code
import time
def api_call_with_retry(api_function, retries=3, delay=5):
for attempt in range(retries):
try:
# Attempt the API call
response = api_function()
return response
except AmazonAPIError as e:
if "Called Too Early" in str(e):
time.sleep(delay)
else:
raise e
raise AmazonAPIError("Max retries reached. Could not complete the request.")
This retry logic allows the system to handle transient errors and ensures that calls are only retried after an appropriate delay.
4. Verify Data Availability Before Making Requests
Ensure that the data you are trying to access is available and ready for retrieval. This is particularly important when dealing with order or inventory data.
- Solution: For APIs that retrieve data from Amazon (like order or inventory APIs), include checks to ensure the requested data is available. For example, when pulling order information, ensure the order has been processed and is ready for retrieval before making the API request.
You can also use APIs like GetReportRequestList to check if the data report you requested has been fully generated before attempting to download it.
5. Use Batch Processing Where Possible
To avoid overloading Amazon’s system with frequent API calls, consider using batch processing. Many Amazon APIs allow you to submit data in bulk, which can prevent unnecessary calls that might trigger the “Called Too Early” error.
- Solution: Instead of sending one API call per product or per order, batch your requests to reduce the number of interactions with Amazon’s servers. This also helps stay within rate limits.
For example, when submitting product listings, use the MWS feed system to submit multiple listings in a single feed rather than submitting them one by one.
Preventing the “Called Too Early” Error
While fixing the error is important, it’s equally crucial to prevent it from occurring in the first place. Here are few preventive metering you can performance:
- Use Amazon’s API Guidelines: Always stay updated with Amazon’s API documentation and follow the recommended best practices for API usage and rate limits.
- Optimize Feed Submission Timing: When dealing with feed submissions or updates, ensure that your system waits for the completion of the current task before initiating a new one. Use API calls to check the status of previous submissions.
- Develop a Monitoring System: Create a monitoring system that tracks API usage and response times. If errors like “Called Too Early” are detected, pause operations and retry after the appropriate delay.
- Consult Amazon Developer Forums: Amazon has active forums and support channels where developers frequently discuss errors and troubleshooting techniques. Staying engaged with these communities can help you stay ahead of common issues.
Conclusion
How to fix called too early Amazon error may seem like a technical hurdle, but it is usually a result of making premature API calls before certain conditions are met. By following the steps outlined above—checking API rate limits, ensuring task completion, implementing retry logic, and optimizing API calls—you can fix and prevent this error from disrupting your Amazon business.
Understanding the causes and best practices for handling this error will help streamline your operations and ensure smoother API interactions, saving both time and resources in the long run.
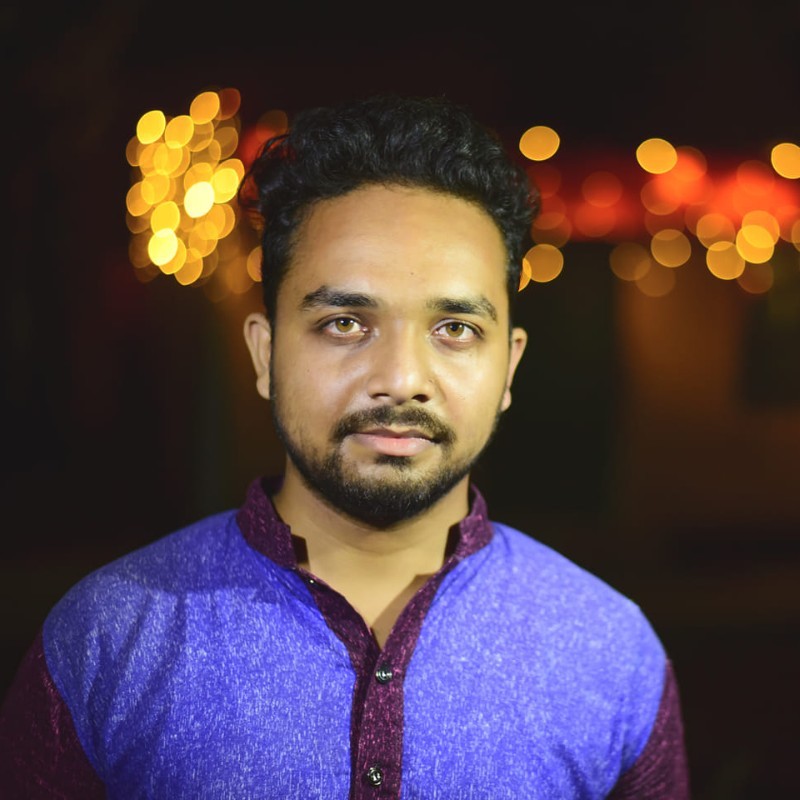
Hello, I am an E-commerce Expert with extensive experience providing services to numerous e-commerce brands and individuals since 2017. My primary areas of expertise include the Amazon, Walmart, and Shopify marketplaces. Linkedin